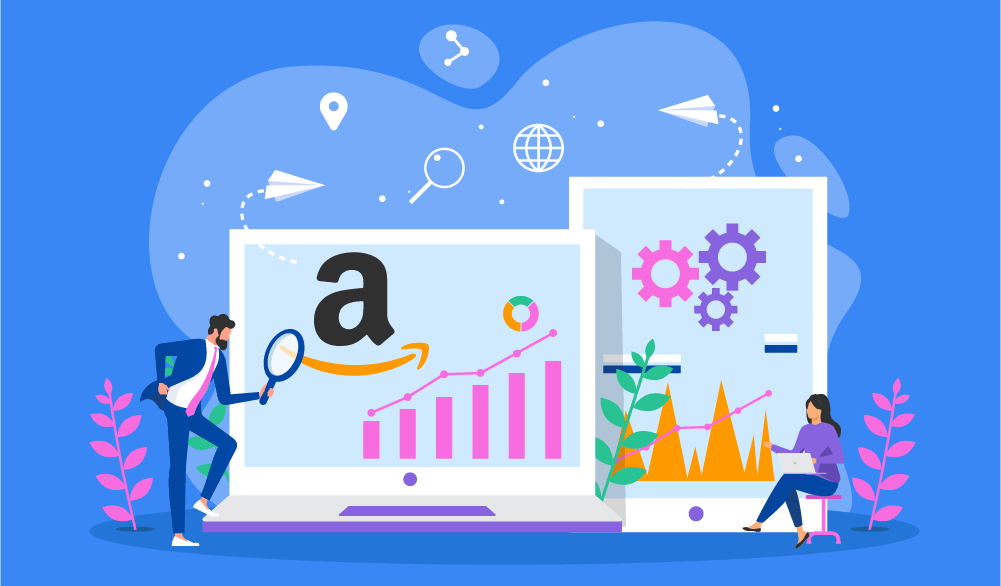
How Can I Scrape Data From Amazon
For many different purposes, including market research, pricing tracking, competitor analysis, and the creation of recommendation systems, scraping data from Amazon can be a highly effective strategy. As one of the biggest online marketplaces, Amazon provides various product information, user feedback, pricing information, etc. However, it’s crucial to remember that obtaining data from Amazon is difficult because the website uses several anti-scraping safeguards to guard its information.
Step 1: Import the necessary libraries
In the first step of scraping data from Amazon, you must import the necessary libraries to provide the required web scraping functionality. The two essential libraries we’ll be using are Beautiful Soup and Requests.
Beautiful Soup is a powerful Python library for parsing HTML and XML documents. It exhibits a simple and intuitive interface for extracting data from HTML or XML files, making it an ideal choice for web scraping tasks. The library allows you to navigate and search through the parsed document using various methods and selectors.
On the other hand, the requests library is a widely-used Python library for sending HTTP requests and handling responses. It simplifies making HTTP requests to web servers and retrieving the content of web pages. You can easily retrieve the HTML content of the Amazon webpage you want to scrape by utilizing requests.
To import these libraries into your Python script, use the following code:
python
from bs4 import BeautifulSoup import requests
In the code snippet above, we import the BeautifulSoup class from the bs4 module, which parses the HTML content. Additionally, we import the requests library to handle the HTTP requests and responses.
By importing these libraries, you ensure you have the tools to parse HTML and send requests to retrieve the webpage content, setting the stage for the subsequent steps in the scraping process.
Step 2: Send a request to the Amazon webpage
Once you have imported the required libraries, the next step is to send an HTTP request to the Amazon webpage you want to scrape. This step allows you to retrieve the HTML content of the webpage, which will be used for further parsing and data extraction.
To send a request, you will use the requests.get() method from the requests library. That will send a GET request to the specified URL and return the server’s response.
python
url = “https://www.amazon.com/” # Replace with the desired Amazon webpage URL response = requests.get(url)
In the code snippet above, we first specify the URL of the Amazon webpage we want to scrape by assigning it to the url variable. You can modify the URL to match your specific scraping requirements.
Next, we use the requests.get() method to send a GET request to the specified URL. The response from the server is stored in the response variable.
By executing these lines of code, you connect to the Amazon webpage and retrieve its HTML content. The response object contains various attributes and methods that allow you to access the server’s response information, such as the status code and content.
It’s important to consider that the success of the request depends on factors such as the web page’s availability, the URL structure, and any additional parameters or headers that might be required for authentication or authorization.
In the next step, we will utilize the obtained HTML content to create a Beautiful Soup object for parsing and extracting data.
Step 3: Create a Beautiful Soup object
After you have obtained the HTML content of the Amazon webpage by sending a request, the next step is creating a Beautiful Soup object. This object will parse the HTML content and provide a structured webpage representation, enabling easy navigation and data extraction.
To create a Beautiful Soup object, import the Beautiful Soup library and pass the HTML content to the BeautifulSoup() constructor.
python
from bs4 import BeautifulSoup # Assuming ‘response’ contains the HTML content from the previous step soup = BeautifulSoup(response.content, “html.parser”)
We import the BeautifulSoup class from the bs4 module in the code snippet above. We then pass the response.content (the HTML content) and the parser type “html.parser” to the BeautifulSoup() constructor, creating a Beautiful Soup object named soup.
The BeautifulSoup() constructor converts the HTML content into a parse tree, representing the HTML document’s hierarchical structure. This parse tree allows you to navigate and search through the HTML elements using various methods and selectors provided by Beautiful Soup.
Creating a Beautiful Soup object gives you access to many powerful methods and properties that simplify extracting data from the HTML content. You can now look forward to the next step, which involves locating the specific HTML elements on the webpage that contain the data you want to scrape.
Step 4: Find the HTML elements containing the data you want to scrape
After creating a Beautiful Soup object and parsing the HTML content of the Amazon webpage, the next step is to locate the HTML elements that contain the specific data you want to scrape. Beautiful Soup provides various methods and selectors to find and extract elements based on their HTML tags, attributes, classes, or other identifying properties.
You can use the find_all() method provided by Beautiful Soup to find the HTML elements. This method allows you to search for all occurrences of a particular HTML element that match the specified criteria.
python
product_titles = soup.find_all(“span”, class_=”a-size-medium a-color-base a-text-normal”) prices = soup.find_all(“span”, class_=”a-price-whole”) ratings = soup.find_all(“span”, class_=”a-icon-alt”)
In the code snippet above, we use the find_all() method to locate the HTML elements that contain the product titles, prices, and ratings on the Amazon webpage.
We specify the desired HTML element using the first argument of the find_all() method, in this case, the “span” tag. We also provide the class attribute as the second argument to narrow the search to elements with specific classes. You can modify the class names or attributes according to the webpage structure.
After executing these lines of code, the product_titles, prices, and rating variables will contain lists of HTML elements that match the specified criteria. These elements are ready for further extraction of the desired data.
In the next step, we will extract the data from these HTML elements to retrieve the product titles, prices, and ratings.
Step 5: Extract the data from the HTML elements
Once you have located the HTML elements with the desired data on the Amazon webpage, extracting the actual information from those elements is next. Beautiful Soup provides various methods and properties to retrieve text, attributes, or other data associated with HTML elements.
In our case, we will focus on extracting the text content of the elements that contain the product titles, prices, and ratings.
python
product_titles_text = [title.get_text() for title in product_titles] prices_text = [price.get_text() for price in prices] ratings_text = [rating.get_text() for rating in ratings]
In the code snippet above, we use list comprehensions to extract the text content of each HTML element. The get_text() method is called on each element, which returns the textual content within the element, excluding any HTML tags or attributes.
By executing these lines of code, we obtain lists (product_titles_text, prices_text, ratings_text) that contain the extracted data in a structured format. Each list element corresponds to the data point extracted from the HTML elements.
You can further process or manipulate this extracted data as per your requirements. For example, you can store it in a database, write it to a file, or perform data analysis tasks.
It’s important to note that the extraction process varies depending on the structure of the webpage and the specific data you are targeting. Adjust the code accordingly to extract the desired information accurately.
Step 6: Print or store the scraped data
After extracting the desired data from the HTML elements, the final step is to decide what to do with the scraped information. You can print it to the console for immediate visibility or store it for further analysis, visualization, or other processing tasks.
Let’s assume we have extracted the product titles, prices, and ratings in the previous steps. Here’s an example of how you can print or store the scraped data:
python
for i in range(len(product_titles_text)): print(“Product:”, product_titles_text[i]) print(“Price:”, prices_text[i]) print(“Rating:”, ratings_text[i]) print()
In the code snippet above, we iterate over the extracted data using a for loop and print each product’s title, price, and rating. The len(product_titles_text) represents the number of items scraped.
You can modify the printing logic to suit your requirements by formatting the output, adding additional information, or writing it to a file. For example, you can write the scraped data to a CSV file with csv module or store it in a database for additional analysis.
If you decide to store the data, consider using appropriate data structures and file formats that best suit your needs. CSV, JSON, or a database (such as SQLite or MySQL) are common options for storing structured data.
Remember to handle any exceptions or errors during the printing or storage and ensure compliance with data protection and scraping policies.
By completing this final step, you have successfully scraped the desired data from the Amazon webpage and have it ready for analysis, visualization, or further processing.
Conclusion
Lastly, web scraping data from Amazon entails importing the required libraries, sending a request to the website, generating a Beautiful Soup object, locating the essential HTML elements, extracting the data, and printing or storing the scraped data. For online scraping projects to be successful and ethical, proper comprehension and adherence to scraping principles are essential.