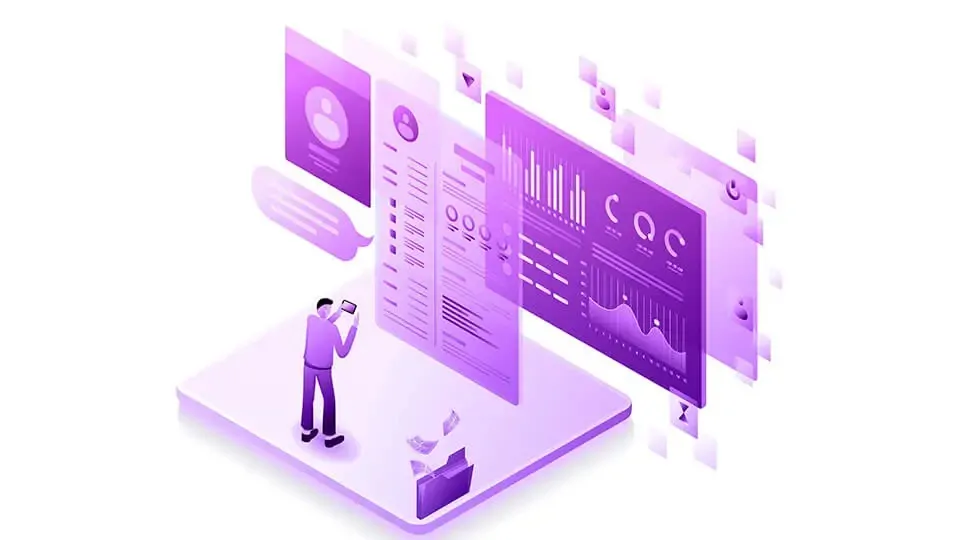
How To Scrape Instagram Posts Using Instagram’s API
Instagram, as one of the most prevalent social media platforms, holds worthwhile data in the form of user-generated content. Enterprises, data scientists, and researchers often look to make use of Instagram data to get a sense of contemporary user behaviours and trending trends. The Application Programming Interface (API) of Instagram provides a structured and lawful way of interacting with the platform, which allows users the fetching data like posts, captions, and user profiles instantly. In contrast to typical scraping measures, getting help from APIs makes sure that you comply with Insta’s terms of service, offering you a more effective approach to accessing useful information. The most distinctive attribute of Instagram API is its capability of data retrieving while guarding privacy and security terms. To work with that API, the users need to pursue a gradual process which revolves around going through the authentification and permission to access specific information. The following steps of this blog will highlight the process of scraping Instagram data using its Graph API in the most possibly simplified and streamlined way.
Step 1: Creating A Facebook Developer Account
The initial step in utilizing Instagram’s API is creating a Facebook Developer account. Proceed over to the Facebook Developer Console and sign in. In case you do not have an account, sign up to begin with. After you are logged in, head to the Developer Dashboard and press on Create App. Select the Instagram or Instagram Basic Display alternative to get commenced. Enter the app title and contact e-mail, and then finalize the creation process.
That will offer you fundamental credentials like the client ID, client secret, and redirect URI. These will all be utilized for OAuth verification. The client ID is utilized to specify your app during API requests. Likewise, the client secret confirms your app’s security as you interact with Instagram’s API.
For instance, you can get help from the following Python snippet to get yourself an access token:
import requests
# OAuth URL for getting access token
url = ‘https://graph.facebook.com/v10.0/oauth/access_token’
params = {
‘client_id’: ‘YOUR_CLIENT_ID’,
‘redirect_uri’: ‘YOUR_REDIRECT_URI’,
‘client_secret’: ‘YOUR_CLIENT_SECRET’,
‘code’: ‘AUTHORIZATION_CODE’
}
response = requests.get(url, params=params)
if response.status_code == 200:
access_token = response.json()[‘access_token’]
print(“Access Token:”, access_token)
else:
print(“Error:”, response.text)
Once you fetch your token, you are now ready to move on to the next step of scraping Instagram posts using Instagram’s API.
Step 2: Creating And Connecting A Business Profile
To approach Instagram’s API, you must make sure that your account is a Business or Creator profile. If it is not already, you can convert your personal Instagram account into a Business or Creator profile through Settings > Account > Switch to Professional Account. Select either Business or Creator based on your essentials.
After that, link your Instagram account to a Facebook page. You can either make a new Facebook page or attach your Instagram account to an existing one. From the Facebook page settings, click on Instagram and press Connect Account. Following that, you will Log in with your Instagram credentials and authorize the fundamental permissions.
After you are done with linking your Instagram account, you can start communicating with Instagram’s API and retrieving media data.
Below is a Python code that will help you in fetching Instagram media:
import requests
# Instagram Graph API URL to get account media
url = ‘https://graph.instagram.com/me/media’
params = {
‘access_token’: ‘YOUR_ACCESS_TOKEN’
}
response = requests.get(url, params=params)
if response.status_code == 200:
data = response.json()
print(“Instagram Media Data:”, data)
else:
print(“Error fetching media:”, response.text)
Step 3: Creating An App Within The Facebook Developer Console
Once you have setup your Instagram Business profile, you will now move on to make an app within the Facebook Developer Console. Proceed to Facebook Developer Console, sign in, and move to My Apps, then click Create App. Select For Everything Else, which functions for Instagram API use.
Input a title for the app, your contact e-mail, and indicate the app’s purpose, then tap on Create App ID. Facebook may ask you to verify, like assembling CAPTCHA.
Once you have created the app, you’ll have access to fundamental credentials like the App ID and App Secret. These credentials empower secure interactions with the Instagram API. The following is an illustration of utilizing these credentials to fetch information from Facebook:
import requests
# Facebook App ID and App Secret
app_id = ‘YOUR_APP_ID’
app_secret = ‘YOUR_APP_SECRET’
# URL to retrieve app data
url = f’https://graph.facebook.com/{app_id}/?access_token={app_id}|{app_secret}’
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print(“App Information:”, data)
else:
print(“Error fetching app data:”, response.text)
Step 4: Creating An Access Token
After setting up and linking your Instagram account, the fourth step is producing an access token. This token will be utilized for verification when making API requests to Instagram.
The most straightforward way is to utilize OAuth 2.0. Develop an OAuth URL with suitable parameters. The user ought to log in and allow your app authorizations to access Instagram information; look into the following example:
https://www.facebook.com/v10.0/dialog/oauth?
client_id=YOUR_CLIENT_ID&
redirect_uri=YOUR_REDIRECT_URI&
state=YOUR_STATE_PARAMETER&
scope=instagram_basic
After the users log in, they are redirected back to the given URI with an authorization code within the URL. Utilize this code to exchange it for an access token. The following is an example of utilizing Python:
import requests
url = ‘https://graph.facebook.com/v10.0/oauth/access_token’
params = {
‘client_id’: ‘YOUR_CLIENT_ID’,
‘client_secret’: ‘YOUR_CLIENT_SECRET’,
‘redirect_uri’: ‘YOUR_REDIRECT_URI’,
‘code’: ‘AUTHORIZATION_CODE’ # The authorization code you got
}
response = requests.get(url, params=params)
if response.status_code == 200:
access_token = response.json()[‘access_token’]
print(“Access Token:”, access_token)
else:
print(“Error fetching access token:”, response.text)
Finally, you will get an access token, which is fundamental for API calls to Instagram’s Graph API.
Step 5: Fetching Instagram Media Data
The fifth step of the scraping process involves Instagram media data, like posts, captions, and pictures. The URL you will utilize to retrieve media is https://graph.instagram.com/me/media.
Create a GET request with the access token as a parameter to retrieve media particulars. You can consider the following Python code for that purpose:
import requests
url = ‘https://graph.instagram.com/me/media’
params = {
‘access_token’: ‘YOUR_ACCESS_TOKEN’
}
response = requests.get(url, params=params)
if response.status_code == 200:
data = response.json()
print(“Instagram Media Data:”, data)
else:
print(“Error fetching media:”, response.text)
It will return media-related data, including media ID, type, caption, and URLs, to approach the media files.
Step 6: Handling Pagination For Vast Data Sets
In case your account includes numerous posts, you might face pagination within the response. Instagram API yields a paging object with a next field that contains the URL for the subsequent set of outcomes. That permits you to proceed with fetching all media information in case there’s a lot to recover.
Look into the following example to help you in handling pagination:
import requests
url = ‘https://graph.instagram.com/me/media’
params = {
‘access_token’: ‘YOUR_ACCESS_TOKEN’
}
while url:
response = requests.get(url, params=params)
if response.status_code == 200:
media_data = response.json()
for media in media_data[‘data’]:
print(f”Media ID: {media[‘id’]}”)
print(f”Media URL: {media[‘media_url’]}”)
print(f”Caption: {media.get(‘caption’, ‘No caption’)}”)
print(‘-‘ * 50)
url = media_data.get(‘paging’, {}).get(‘next’)
else:
print(“Error fetching media:”, response.text)
break
The above code will proceed to request additional pages till retrieving all media data.
Step 7: Integrating The Data Into Your Project
In this final step, you’ll integrate the media data into your project. Whether you are constructing a website analysis tool or an app, the fetched media information can be portrayed or utilized for further processing. You can also store this information in a database or depict it on a webpage.
Below is an example to help you to store it as a CSV file:
import csv
with open(‘instagram_media.csv’, mode=’w’, newline=”) as file:
writer = csv.writer(file)
writer.writerow([“Media ID”, “Media URL”, “Caption”])
for media in media_data[‘data’]:
writer.writerow([media[‘id’], media[‘media_url’], media.get(‘caption’, ‘No caption’)])
On saving the data, you can now reference and process Instagram posts offline or display them afterwards. Whether showing images, captions, or analyzing post-performance, the adaptability of Instagram’s Graph API faciliattaes you with a number of capabilities.
The steps mentioned in this blog post enable you to utilize Instagram data consistently in your applications.
Conclusion
In conclusion, Instagram data scraping can yield insightful information for market research via its user-generated data. Businesses may gain a better understanding of their target consumers by analyzing user behaviours, preferences, patterns, and interaction patterns. Additionally, Instagram’s Graph API helps organizations find notable users, popular hashtags, and trending material. Instagram data can also be useful to researchers and academics investigating social media behaviour, cultural trends, user psychology, and other pertinent issues, providing large-scale datasets for analysis and better comprehension. To put it briefly, users can use the Instagram data that its Graph API provides for a variety of applications.