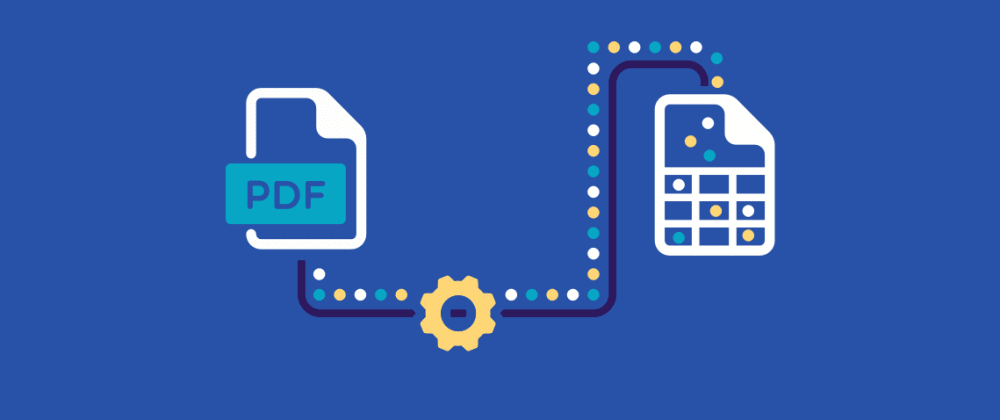
How To Use Apache PDFBox For Data Extraction From PDFs
PDF is a prevalent document publishing format since users see it as a digital substitute for paper documents. Yet when information, especially structured data, is enclosed within a PDF document, and you want to extract that content, this format becomes quite difficult for users to interact with. Extracting text from PDF documents is a frequent task in various fields, especially for data processing, document management, and information retrieval systems. Generally, parsing is considered a useful approach when dealing with text-based PDFs as it is swift and more accurate. When looking for a suitable tool to perform this kind of data extraction, Apache PDFBox, which is a popular open-source Java library, becomes a perfect alternative to parse PDFs efficiently. It aids developers in creating, manipulating, and extracting data from PDF documents. Apache PDFBox holds a powerful set of features for handling PDF files, including creating new PDFs, adding content, extracting text, and more. The following steps of this blog post will further elaborate thoroughly on extracting useful data from PDF files using Apache PDFBox.
Step 1: Setting Up The Apache PDFBox Library In Your Project
In case you are using Java, the only way to do that is to include PDFBox as a dependency through a build tool such as Maven or Gradle. For Maven, you’ll incorporate the below-mentioned dependency in your pom.xml file:
<dependency>s
<groupId>org.apache.pdfbox</groupId>
<artifactId>pdfbox</artifactId>
<version>2.0.27</version>
</dependency>
On the off chance that you are utilizing Gradle, here is an illustration of it:
implementation ‘org.apache.pdfbox:pdfbox:2.0.27’
After the library is incorporated into your project, you will have access to all the fundamental classes and strategies required for PDF manipulation. In case you’re not employing a build tool, you’ll be able to download the Jar file specifically from the Apache PDFBox site and manually incorporate it into the classpath of your project.
Once you are done with setting up PDFBox, make sure that your development environment is accurately arranged to recognize the library. Once everything is in order, you’re all set to begin composing code to load and extract content from PDFs. The beginning layout is basic to getting to PDFBox’s effective PDF parsing and data extraction highlights. After it is executed, you can proceed to the second phase for actual information extraction from PDF files.
Step 2: Loading The PDF Document
This step involves loading the PDF document that you need to extract data from. It will be accomplished utilizing the PDDocument.load() strategy, which takes in a File object defining the PDF. The following is an illustration of doing it in your Java code:
File pdfFile = new File(“path/to/your/document.pdf”);
PDDocument document = PDDocument.load(pdfFile);
This strategy will open the PDF and load it into a PDDocument object. This is imperative to handle exceptions at this phase, as the PDF could be corrupt or incoherent. The PDDocument.load() strategy can throw an IOException in the event that there is a problem with unlocking the file, so it is most reasonable to wrap the loading process in a try-catch block.
After the document is loaded effectively, you now have access to the PDF’s content and can commence with extracting data from it. Be sure to shut the PDDocument once you are done to release system resources by calling document.close(). It guarantees proficient memory management, especially when working with expansive PDF files.
Once the document is loaded, you are prepared to head to the third step to extract the content and data it retains.
Step 3: Extracting The Text Content From The PDF Document
The third step involves extracting the text content from the PDF document. Apache PDFBox offers the PDFTextStripper class for that pursuit.
That class permits you to extract plain content from all or particular pages of the document. To start, you will instantiate a PDFTextStripper object and call the getText() strategy on the document to recover the text. To extract the text from an entire document, use this:
PDFTextStripper stripper = new PDFTextStripper();
String text = stripper.getText(document);
System.out.println(text);
If you need to extract text from particular pages, you’ll set the start and end page utilizing the setStartPage() and setEndPage() strategies before calling getText():
stripper.setStartPage(1);
stripper.setEndPage(5);
String text = stripper.getText(document);
System.out.println(text);
That approach will permit for more focused extraction, particularly if you only require information from particular pages of a huge document. Be sure that the quality of text extraction relies on the PDF’s layout; if it is scanned or comprises pictures, the text may not be extracted precisely. For such possibilities, you might have to utilize OCR strategies besides PDFBox. Once the text is extracted, you’ll be able to continue to examine or parse it for the particular data you need.
Step 4: Extracting Content From Specific Page Ranges
When managing an expansive PDF or one that comprises data spread over numerous pages, it can often be useful to extract content from specific page ranges rather than preparing the whole document. Apache PDFBox’s PDFTextStripper class permits you to define the range of pages from which you need to extract content.
To indicate a page range, you’ll utilize the setStartPage() and setEndPage() strategies on the PDFTextStripper instance. These strategies permit you to define the initial and last pages that will be processed, offering you control over the content extraction process.
For example, in case you only need to extract text from pages 3 to 5, you can do the following:
PDFTextStripper stripper = new PDFTextStripper();
stripper.setStartPage(3);
stripper.setEndPage(5);
String text = stripper.getText(document);
System.out.println(text);
This way, it will be ensured that the text of pages 3 to 5 is extracted, making it more straightforward for documents that have unnecessary pages. It will moreover save your time and minimize the memory usage.
Step 5: Parsing And Extracting The Particular Data
When it comes to parsing data of the documents containing tables, addresses or any other structured format, you need to utilize regex or standard expressions. It will help in locating the patterns that conform to your desired data.
For instance, to extract all e-mail addresses from the content, you will utilize a regex pattern that approximates the standard e-mail formats. Here is an example:
Pattern emailPattern = Pattern.compile(“[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,}”);
Matcher matcher = emailPattern.matcher(text);
while (matcher.find()) {
System.out.println(matcher.group());
}
Consider splitting up the text into small parts to process overly structured and complex data smoothly; you can do filtering of unnecessary components, address special characters or even employ NLP tools.
Step 6: Saving Or Exporting The Data In A Desired Format
If you want the CSV file as your preferred format to save the data, you will have to write the data line by line via Java FileWriter. Here is an example:
import java.io.FileWriter;
import java.io.IOException;
try (FileWriter writer = new FileWriter(“extracted_data.csv”)) {
writer.append(“Email\n”); // Header for the CSV file
while (matcher.find()) {
writer.append(matcher.group()).append(“\n”); // Write each extracted email
}
} catch (IOException e) {
e.printStackTrace();
}
In case your extracted information is more complex, like nested data, you may favour a JSON format. The org.json library can aid in transforming your information into a JSON object and saving it to a file:
import org.json.JSONObject;
import java.io.FileWriter;
import java.io.IOException;
JSONObject jsonObject = new JSONObject();
jsonObject.put(“emails”, emailList);
try (FileWriter file = new FileWriter(“extracted_data.json”)) {
file.write(jsonObject.toString());
} catch (IOException e) {
e.printStackTrace();
}
Similarly, in the case of larger data sets, you might store the extracted data in a relational database or a NoSQL database, per the data configuration. It will guarantee that you can query and control the data efficiently.
Regardless of which format you select, saving the extracted information properly guarantees that you can access, examine, or share it later, making the whole extraction process more valuable and organised.
Conclusion:
To conclude, for developers, researchers, and organisations alike, extracting data from PDF documents poses a series of difficulties that might make the task more difficult. Even though PDFS are widely used for digital documents, data extraction attempts are made more difficult by their inherent features and variety of formats. Nevertheless, organizations can overcome the inherent challenges of extracting data from PDFs and uncover significant insights by using the appropriate tools and procedures. Correspondingly, Apache PDFBox is a useful tool that is recommended for this purpose. It assists developers in a variety of PDF-related activities, including report generation, form processing, and, most importantly, PDF data extraction.